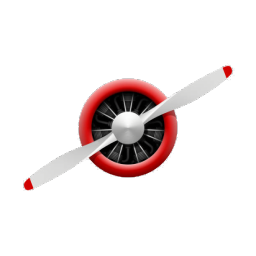 |
AirControl
1.3.0
Open Source, Modular, and Extensible Flight Simulator For Deep Learning Research
|
9 using System.Collections.Generic;
12 using UnityEngine.EventSystems;
28 get {
return m_ChartName; }
31 if (!
string.IsNullOrEmpty(value) &&
XChartsMgr.Instance.ContainsChart(value))
33 Debug.LogError(
"chartName repeated:" + value);
49 public Title title {
get {
return m_Titles.Count > 0 ? m_Titles[0] :
null; } }
50 public List<Title> titles {
get {
return m_Titles; } }
55 public Legend legend {
get {
return m_Legends.Count > 0 ? m_Legends[0] :
null; } }
56 public List<Legend> legends {
get {
return m_Legends; } }
61 public Tooltip tooltip {
get {
return m_Tooltips.Count > 0 ? m_Tooltips[0] :
null; } }
76 public DataZoom dataZoom {
get {
return m_DataZooms.Count > 0 ? m_DataZooms[0] :
null; } }
77 public List<DataZoom> dataZooms {
get {
return m_DataZooms; } }
83 public List<VisualMap> visualMaps {
get {
return m_VisualMaps; } }
88 public float chartX {
get {
return m_ChartX; } }
93 public float chartY {
get {
return m_ChartY; } }
104 public Vector2 chartMinAnchor {
get {
return m_ChartMinAnchor; } }
105 public Vector2 chartMaxAnchor {
get {
return m_ChartMaxAnchor; } }
106 public Vector2 chartPivot {
get {
return m_ChartPivot; } }
107 public Vector2 chartSizeDelta {
get {
return m_ChartSizeDelta; } }
113 public Rect chartRect {
get {
return m_ChartRect; } }
117 public Action<VertexHelper>
onCustomDraw {
set { m_OnCustomDrawBaseCallback = value; } }
129 public Action<VertexHelper>
onCustomDrawTop {
set { m_OnCustomDrawTopCallback = value; } }
134 public Action<PointerEventData, int, int>
onPointerClickPie {
set { m_OnPointerClickPie = value; m_ForceOpenRaycastTarget =
true; }
get {
return m_OnPointerClickPie; } }
142 m_RefreshChart =
true;
143 if (m_Painter) m_Painter.Refresh();
156 m_CheckAnimation =
false;
157 m_ReinitLabel =
true;
169 foreach (var radar
in m_Radars) radar.indicatorList.Clear();
172 m_CheckAnimation =
false;
173 m_ReinitLabel =
true;
174 m_SerieLabelRoot =
null;
187 m_SerieLabelRoot =
null;
201 return m_Series.
AddSerie(type, serieName, show, addToHead);
212 public virtual Serie AddSerie(
string serieType,
string serieName =
null,
bool show =
true,
bool addToHead =
false)
215 var list = Enum.GetNames(typeof(
SerieType));
216 foreach (var t
in list)
220 return AddSerie(type, serieName, show, addToHead);
223 public virtual Serie InsertSerie(
int index,
SerieType serieType,
string serieName =
null,
bool show =
true)
225 return m_Series.InsertSerie(index, serieType, serieName, show);
239 var serieData = m_Series.
AddData(serieName, data, dataName);
240 if (serieData !=
null)
242 var serie = m_Series.
GetSerie(serieName);
243 if (SerieHelper.GetSerieLabel(serie, serieData).show)
247 RefreshPainter(serie);
262 var serieData = m_Series.
AddData(serieIndex, data, dataName);
263 if (serieData !=
null)
265 var serie = m_Series.
GetSerie(serieIndex);
266 if (SerieHelper.GetSerieLabel(serie, serieData).show)
270 RefreshPainter(serie);
283 public virtual SerieData AddData(
string serieName, List<double> multidimensionalData,
string dataName =
null)
285 var serieData = m_Series.
AddData(serieName, multidimensionalData, dataName);
286 if (serieData !=
null)
288 var serie = m_Series.
GetSerie(serieName);
289 if (SerieHelper.GetSerieLabel(serie, serieData).show)
293 RefreshPainter(serie);
306 public virtual SerieData AddData(
int serieIndex, List<double> multidimensionalData,
string dataName =
null)
308 var serieData = m_Series.
AddData(serieIndex, multidimensionalData, dataName);
309 if (serieData !=
null)
311 var serie = m_Series.
GetSerie(serieIndex);
312 if (SerieHelper.GetSerieLabel(serie, serieData).show)
316 RefreshPainter(serie);
330 public virtual SerieData AddData(
string serieName,
double xValue,
double yValue,
string dataName =
null)
332 var serieData = m_Series.
AddXYData(serieName, xValue, yValue, dataName);
333 if (serieData !=
null)
335 var serie = m_Series.
GetSerie(serieName);
336 if (SerieHelper.GetSerieLabel(serie, serieData).show)
340 RefreshPainter(serie);
354 public virtual SerieData AddData(
int serieIndex,
double xValue,
double yValue,
string dataName =
null)
356 var serieData = m_Series.
AddXYData(serieIndex, xValue, yValue, dataName);
357 if (serieData !=
null)
359 var serie = m_Series.
GetSerie(serieIndex);
360 if (SerieHelper.GetSerieLabel(serie, serieData).show)
364 RefreshPainter(serie);
368 public virtual SerieData AddData(
int serieIndex,
double open,
double close,
double lowest,
double heighest,
string dataName =
null)
370 var serieData = m_Series.
AddData(serieIndex, open, close, lowest, heighest, dataName);
371 if (serieData !=
null)
373 var serie = m_Series.
GetSerie(serieIndex);
374 if (SerieHelper.GetSerieLabel(serie, serieData).show)
378 RefreshPainter(serie);
390 public virtual bool UpdateData(
string serieName,
int dataIndex,
double value)
392 if (m_Series.
UpdateData(serieName, dataIndex, value))
394 RefreshPainter(m_Series.
GetSerie(serieName));
407 public virtual bool UpdateData(
int serieIndex,
int dataIndex,
double value)
409 if (m_Series.
UpdateData(serieIndex, dataIndex, value))
411 RefreshPainter(m_Series.
GetSerie(serieIndex));
423 public virtual bool UpdateData(
string serieName,
int dataIndex, List<double> multidimensionalData)
425 if (m_Series.
UpdateData(serieName, dataIndex, multidimensionalData))
427 RefreshPainter(m_Series.
GetSerie(serieName));
439 public virtual bool UpdateData(
int serieIndex,
int dataIndex, List<double> multidimensionalData)
441 if (m_Series.
UpdateData(serieIndex, dataIndex, multidimensionalData))
443 RefreshPainter(m_Series.
GetSerie(serieIndex));
456 public virtual bool UpdateData(
string serieName,
int dataIndex,
int dimension,
double value)
458 if (m_Series.
UpdateData(serieName, dataIndex, dimension, value))
460 RefreshPainter(m_Series.
GetSerie(serieName));
473 public virtual bool UpdateData(
int serieIndex,
int dataIndex,
int dimension,
double value)
475 if (m_Series.
UpdateData(serieIndex, dataIndex, dimension, value))
477 RefreshPainter(m_Series.
GetSerie(serieIndex));
490 public virtual bool UpdateDataName(
string serieName,
int dataIndex,
string dataName)
502 public virtual bool UpdateDataName(
int serieIndex,
int dataIndex,
string dataName)
513 public virtual void SetActive(
string serieName,
bool active)
515 var serie = m_Series.
GetSerie(serieName);
528 public virtual void SetActive(
int serieIndex,
bool active)
531 var serie = m_Series.
GetSerie(serieIndex);
532 if (serie !=
null && !
string.IsNullOrEmpty(serie.name))
534 UpdateLegendColor(serie.name, active);
538 public virtual void UpdateLegendColor(
string legendName,
bool active)
540 var legendIndex = m_LegendRealShowName.IndexOf(legendName);
541 if (legendIndex >= 0)
543 foreach (var
legend in m_Legends)
545 var iconColor = LegendHelper.GetIconColor(
this, legendIndex, legendName, active);
546 var contentColor = LegendHelper.GetContentColor(legendIndex,
legend, m_Theme, active);
561 return m_Series.
IsActive(serieName);
572 return m_Series.
IsActive(serieIndex);
583 foreach (var serie
in m_Series.
list)
585 if (serie.show && legendName.Equals(serie.name))
591 foreach (var serieData
in serie.data)
593 if (serieData.show && legendName.Equals(serieData.name))
609 m_ReinitLabel =
true;
610 m_SerieLabelRoot =
null;
630 Debug.LogError(
"UpdateTheme: not support switch to Custom theme.");
644 m_Theme.CopyTheme(
theme);
645 SetAllComponentDirty();
647 UnityEditor.EditorUtility.SetDirty(
this);
658 foreach (var serie
in m_Series.
list) serie.AnimationEnable(flag);
667 foreach (var serie
in m_Series.
list) serie.AnimationFadeIn();
676 foreach (var serie
in m_Series.
list) serie.AnimationFadeOut();
685 foreach (var serie
in m_Series.
list) serie.AnimationPause();
694 foreach (var serie
in m_Series.
list) serie.AnimationResume();
703 foreach (var serie
in m_Series.
list) serie.AnimationReset();
714 OnLegendButtonClick(legendIndex, legendName, show);
730 if (x < m_ChartX || x > m_ChartX + m_ChartWidth ||
731 y < m_ChartY || y > m_ChartY + m_ChartHeight)
738 public void ClampInChart(ref Vector3 pos)
742 if (pos.x < m_ChartX) pos.x = m_ChartX;
743 if (pos.x > m_ChartX + m_ChartWidth) pos.x = m_ChartX + m_ChartWidth;
744 if (pos.y < m_ChartY) pos.y = m_ChartY;
745 if (pos.y > m_ChartY + m_ChartHeight) pos.y = m_ChartY + m_ChartHeight;
749 public Vector3 GetTitlePosition(Title
title)
754 public bool ContainsSerie(
SerieType serieType)
756 return SeriesHelper.ContainsSerie(m_Series, serieType);
759 public virtual bool AddDefaultCustomSerie(
string serieName,
int dataCount = 5)
764 public virtual string[] GetCustomSerieInspectorShowFileds()
768 public virtual string[][] GetCustomSerieInspectorCustomFileds()
772 public virtual string[] GetCustomChartInspectorShowFileds()
777 public virtual string GetCustomSerieTypeName()
782 public virtual bool GetCustomSerieDataNameForColor()
787 public int GetLegendRealShowNameIndex(
string name)
789 return m_LegendRealShowName.IndexOf(name);
792 public virtual void InitCustomSerieTooltip(ref StringBuilder stringBuilder, Serie serie,
int index)
803 if (m_Painter !=
null)
805 m_Painter.material = material;
816 if (m_PainterList !=
null)
818 foreach (var painter
in m_PainterList)
819 painter.material = material;
830 if (m_PainterTop !=
null)
832 m_PainterTop.material = material;
void AnimationResume()
Stop play animation. 继续动画。
Vector3 GetPosition(float chartWidth, float chartHeight)
返回在坐标系中的具体位置
virtual void ClearData()
Remove all series and legend data. It just emptying all of serie's data without emptying the list of ...
void SetBasePainterMaterial(Material material)
设置Base Painter的材质球
void UpdateButtonColor(string name, Color color)
Update the legend button color. 更新图例按钮颜色。
Location location
The location of title component. 标题显示位置。
virtual bool UpdateData(string serieName, int dataIndex, int dimension, double value)
更新指定系列指定索引指定维数的数据。维数从0开始。
VisualMap component. Mapping data to visual elements such as colors. 视觉映射组件。用于进行『视觉编码』,也就是将数据映射到视觉元素(...
SerieData AddData(string serieName, double value, string dataName=null)
添加一个数据到指定系列的维度Y数据中
virtual SerieData AddData(int serieIndex, double xValue, double yValue, string dataName=null)
Add a (x,y) data to serie. 添加(x,y)数据到指定系列中。
virtual SerieData AddData(string serieName, double data, string dataName=null)
Add a data to serie. If serieName doesn't exist in legend,will be add to legend. 添加一个数据到指定的系列中。
void AnimationEnable(bool flag)
Whether series animation enabel. 启用或关闭起始动画。
DataZoom component is used for zooming a specific area, which enables user to investigate data in det...
void RemoveAll()
Remove all serie from series. 移除所有系列。
void ClickLegendButton(int legendIndex, string legendName, bool show)
点击图例按钮
Serie AddSerie(SerieType type, string serieName, bool show=true, bool addToHead=false)
添加一个系列到列表中。
float chartX
The x of chart. 图表的X
SerieType
the type of serie. 系列类型。
void SetActive(string name, bool active)
设置指定系列是否显示
void ClearData()
Clear legend data. 清空。
Title component, including main title and subtitle. 标题组件,包含主标题和副标题。
void UpdateTheme(ChartTheme theme)
Update chart theme info. 切换图表主题。
virtual bool IsActive(int serieIndex)
Whether serie is activated. 获取指定系列是否显示。
void RefreshTooltip()
刷新Tooltip组件。
Tooltip? tooltip
The tooltip setting of chart. 提示框组件
virtual Serie AddSerie(string serieType, string serieName=null, bool show=true, bool addToHead=false)
Add a serie to serie list. 通过字符串类型的serieType添加一个系列到系列列表中。如果serieType不是已定义的SerieType类型,则设置为Custom类型。
bool UpdateTheme(Theme theme)
Update chart theme. 切换内置主题。
virtual bool UpdateDataName(int serieIndex, int dataIndex, string dataName)
Update serie data name. 更新指定系列中的指定索引数据名称。
virtual bool UpdateData(int serieIndex, int dataIndex, int dimension, double value)
更新指定系列指定索引指定维数的数据。维数从0开始。
virtual bool UpdateDataName(string serieName, int dataIndex, string dataName)
Update serie data name. 更新指定系列中的指定索引数据名称。
bool IsInChart(Vector2 local)
坐标是否在图表范围内
DataZoom? dataZoom
dataZoom component. 区域缩放组件。
Settings settings
Global parameter setting component. 全局设置组件。
virtual bool UpdateData(int serieIndex, int dataIndex, double value)
Update serie data by serie index. 更新指定系列中的指定索引数据。
virtual void SetActive(int serieIndex, bool active)
Whether to show serie. 设置指定系列是否显示。
void RefreshChart()
Redraw chart in next frame. 在下一帧刷新图表。
Legend component.The legend component shows different sets of tags, colors, and names....
virtual void SetActive(string serieName, bool active)
Whether to show serie. 设置指定系列是否显示。
bool UpdateDataName(string serieName, int dataIndex, string dataName)
更新指定系列的数据项名称
virtual bool IsActive(string serieName)
Whether serie is activated. 获取指定系列是否显示。
Theme theme
the theme of chart. 主题类型。
virtual SerieData AddData(string serieName, List< double > multidimensionalData, string dataName=null)
Add an arbitray dimension data to serie,such as (x,y,z,...). 添加多维数据(x,y,z...)到指定的系列中。
Action< VertexHelper > onCustomDrawTop
自定义Top绘制回调。在绘制Tooltip前调用。
float chartY
The y of chart. 图表的Y
virtual SerieData AddData(string serieName, double xValue, double yValue, string dataName=null)
Add a (x,y) data to serie. 添加(x,y)数据到指定系列中。
virtual bool UpdateData(string serieName, int dataIndex, double value)
Update serie data by serie name. 更新指定系列中的指定索引数据。
void ClearData()
清空所有系列的数据
Serie GetSerie(string name)
获得指定系列名的第一个系列
List< Serie > list
the list of serie 系列列表。
void AnimationFadeOut()
fadeIn animation. 开始渐出动画。
Series series
The series setting of chart. 系列列表
void SetSeriePainterMaterial(Material material)
设置Serie Painter的材质球
系列。每个系列通过 type 决定自己的图表类型。
the list of series. 系列列表。每个系列通过 type 决定自己的图表类型。
Action< VertexHelper, Serie > onCustomDrawAfterSerie
自定义Serie绘制回调。在每个Serie绘制完后调用。
bool UpdateData(string serieName, int dataIndex, double value)
更新指定系列的维度Y数据
virtual SerieData AddData(int serieIndex, double data, string dataName=null)
Add a data to serie. 添加一个数据到指定的系列中。
Action< VertexHelper, Serie > onCustomDrawBeforeSerie
自定义Serie绘制回调。在每个Serie绘制完前调用。
float chartHeight
The height of chart. 图表的高
virtual Serie AddSerie(SerieType type, string serieName=null, bool show=true, bool addToHead=false)
Add a serie to serie list. 添加一个系列到系列列表中。
void RefreshLabel()
刷新文本标签Label,重新初始化,当有改动Label参数时手动调用改接口
Material topPainterMaterial
Top Pointer 材质球,设置后会影响Tooltip等。
float chartWidth
The width of chart. 图表的宽
virtual void RemoveData(string serieName)
Remove legend and serie by name. 清除指定系列名称的数据。
Legend? legend
The legend setting of chart. 图例组件
SerieData AddXYData(string serieName, double xValue, double yValue, string dataName=null)
添加(x,y)数据到指定的系列中
@ Rect
正方形。可通过设置itemStyle的cornerRadius变成圆角矩形。
virtual SerieData AddData(int serieIndex, List< double > multidimensionalData, string dataName=null)
Add an arbitray dimension data to serie,such as (x,y,z,...). 添加多维数据(x,y,z...)到指定的系列中。
virtual bool UpdateData(string serieName, int dataIndex, List< double > multidimensionalData)
更新指定系列指定索引的数据项的多维数据。
virtual bool UpdateData(int serieIndex, int dataIndex, List< double > multidimensionalData)
更新指定系列指定索引的数据项的多维数据。
void RemoveData(string name)
Removes the legend with the specified name. 移除指定名字的图例。
Action< VertexHelper > onCustomDraw
自定义绘制回调。在绘制Serie前调用。
Material basePainterMaterial
Base Pointer 材质球,设置后会影响Axis等。
void SetTopPainterMaterial(Material material)
设置Top Painter的材质球
string chartName
The name of chart.
bool RemoveSerie(string serieName)
Remove serie from series. 移除指定名字的系列。
void UpdateContentColor(string name, Color color)
Update the text color of legend. 更新图例文字颜色。
Action< PointerEventData, int, int > onPointerClickPie
the callback function of click pie area. 点击饼图区域回调。参数:PointerEventData,SerieIndex,SerieDataIndex
The base class of all charts. 所有Chart的基类。
Title? title
The title setting of chart. 标题组件
void AnimationFadeIn()
fadeIn animation. 开始渐入动画。
virtual bool IsActiveByLegend(string legendName)
Whether serie is activated. 获得指定图例名字的系列是否显示。
void AnimationPause()
Pause animation. 暂停动画。
bool IsActive(string name)
指定系列是否显示
Global parameter setting component. The default value can be used in general, and can be adjusted whe...
VisualMap? visualMap
visualMap component. 视觉映射组件。
virtual void RemoveData()
Remove all data from series and legend. The series list is also cleared. 清除所有系列和图例数据,系列的列表也会被清除。
ChartTheme theme
The theme.
Vector3 chartPosition
The position of chart. 图表的左下角起始坐标。
void AnimationReset()
Reset animation. 重置动画。
A data item of serie. 系列中的一个数据项。可存储数据名和1-n维的数据。