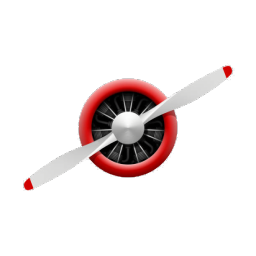 |
AirControl
1.3.0
Open Source, Modular, and Extensible Flight Simulator For Deep Learning Research
|
8 using System.Collections.Generic;
72 [SerializeField]
private bool m_Show =
true;
73 [SerializeField]
private Type m_IconType;
75 [SerializeField]
private Orient m_Orient =
Orient.Horizonal;
77 [SerializeField]
private float m_ItemWidth = 25.0f;
78 [SerializeField]
private float m_ItemHeight = 12.0f;
79 [SerializeField]
private float m_ItemGap = 10f;
80 [SerializeField]
private bool m_ItemAutoColor =
true;
81 [SerializeField]
private bool m_TextAutoColor =
false;
82 [SerializeField]
private string m_Formatter;
84 [SerializeField]
private List<string> m_Data =
new List<string>();
85 [SerializeField]
private List<Sprite> m_Icons =
new List<Sprite>();
87 private Dictionary<string, LegendItem> m_DataBtnList =
new Dictionary<string, LegendItem>();
88 private Dictionary<int, float> m_RuntimeEachWidth =
new Dictionary<int, float>();
96 get {
return m_Show; }
97 set {
if (PropertyUtil.SetStruct(ref m_Show, value)) SetComponentDirty(); }
106 get {
return m_IconType; }
107 set {
if (PropertyUtil.SetStruct(ref m_IconType, value)) SetAllDirty(); }
116 get {
return m_SelectedMode; }
117 set {
if (PropertyUtil.SetStruct(ref m_SelectedMode, value)) SetComponentDirty(); }
126 get {
return m_Orient; }
127 set {
if (PropertyUtil.SetStruct(ref m_Orient, value)) SetComponentDirty(); }
136 get {
return m_Location; }
137 set {
if (PropertyUtil.SetClass(ref m_Location, value)) SetComponentDirty(); }
146 get {
return m_ItemWidth; }
147 set {
if (PropertyUtil.SetStruct(ref m_ItemWidth, value)) SetComponentDirty(); }
156 get {
return m_ItemHeight; }
157 set {
if (PropertyUtil.SetStruct(ref m_ItemHeight, value)) SetComponentDirty(); }
166 get {
return m_ItemGap; }
167 set {
if (PropertyUtil.SetStruct(ref m_ItemGap, value)) SetComponentDirty(); }
176 get {
return m_ItemAutoColor; }
177 set {
if (PropertyUtil.SetStruct(ref m_ItemAutoColor, value)) SetComponentDirty(); }
186 get {
return m_TextAutoColor; }
187 set {
if (PropertyUtil.SetStruct(ref m_TextAutoColor, value)) SetComponentDirty(); }
197 get {
return m_Formatter; }
198 set {
if (PropertyUtil.SetClass(ref m_Formatter, value)) SetComponentDirty(); }
206 get {
return m_TextStyle; }
207 set {
if (PropertyUtil.SetClass(ref m_TextStyle, value)) SetComponentDirty(); }
216 public List<string>
data
218 get {
return m_Data; }
219 set {
if (value !=
null) { m_Data = value; SetComponentDirty(); } }
224 public List<Sprite>
icons
226 get {
return m_Icons; }
227 set {
if (value !=
null) { m_Icons = value; SetComponentDirty(); } }
229 public int index {
get;
internal set; }
242 public override void ClearComponentDirty()
244 base.ClearComponentDirty();
254 public Dictionary<string, LegendItem>
buttonList {
get {
return m_DataBtnList; } }
281 m_IconType =
Type.Auto,
284 m_Orient =
Orient.Horizonal,
287 m_ItemHeight = 12.0f,
290 legend.location.
top = 35;
291 legend.textStyle.offset =
new Vector2(2, 0);
292 legend.textStyle.fontSize = 0;
315 return m_Data.Contains(name);
325 if (m_Data.Contains(name))
339 if (!m_Data.Contains(name) && !
string.IsNullOrEmpty(name))
354 if (index >= 0 && index < m_Data.Count)
356 return m_Data[index];
369 return m_Data.IndexOf(legendName);
378 m_DataBtnList.Clear();
390 m_DataBtnList[name] = item;
391 int index = m_DataBtnList.Values.Count;
393 item.SetActive(
show);
404 if (m_DataBtnList.ContainsKey(name))
406 m_DataBtnList[name].SetIconColor(color);
418 if (m_DataBtnList.ContainsKey(name))
420 m_DataBtnList[name].SetContentColor(color);
432 if (index >= 0 && index < m_Icons.Count)
434 return m_Icons[index];
458 if (
string.IsNullOrEmpty(m_Formatter))
462 var content = m_Formatter.Replace(
"{name}", category);
463 content = content.Replace(
"\\n",
"\n");
464 content = content.Replace(
"<br/>",
"\n");
Orient
the layout is horizontal or vertical. 垂直还是水平布局方式。
List< Sprite > icons
自定义的图例标记图形。
@ Single
单圈雷达图。此时一个雷达只能绘制一个圈,多个serieData组成一个圈,数据取自data[1]。
SelectedMode
Selected mode of legend, which controls whether series can be toggled displaying by clicking legends....
List< string > data
Data array of legend. An array item is usually a name representing string. (If it is a pie chart,...
@ Multiple
多圈雷达图。此时可一个雷达里绘制多个圈,一个serieData就可组成一个圈(多维数据)。
string GetData(int index)
Gets the legend for the specified index. 获得指定索引的图例。
void UpdateButtonColor(string name, Color color)
Update the legend button color. 更新图例按钮颜色。
Dictionary< int, float > runtimeEachWidth
多列时每列的宽度
override bool componentDirty
组件是否需要刷新
float runtimeWidth
运行时图例的总宽度
Location type. Quick to set the general location. 位置类型。通过Align快速设置大体位置,再通过left,right,top,bottom微调具体位置...
string GetFormatterContent(string category)
获得图例格式化后的显示内容。
void ClearData()
Clear legend data. 清空。
float itemGap
The distance between each legend, horizontal distance in horizontal layout, and vertical distance in ...
bool ContainsData(string name)
Whether include in legend data by the specified name. 是否包括由指定名字的图例
Settings related to text. 文本的相关设置。
bool textAutoColor
Whether the legend text matches the color automatically. 图例标记的文本是否自动匹配颜色。 [default:false]
@ None
Don't show as Nightingale chart.不展示成南丁格尔玫瑰图
virtual bool componentDirty
组件重新初始化标记。
Legend component.The legend component shows different sets of tags, colors, and names....
void AddData(string name)
Add legend data. 添加图例。
SelectedMode selectedMode
Selected mode of legend, which controls whether series can be toggled displaying by clicking legends....
bool show
Whether to show legend component. 是否显示图例组件。
Location location
The location of legend. 图例显示的位置。 [default:Location.defaultTop]
float top
Distance between component and the left side of the container. 离容器上侧的距离。
Orient orient
Specify whether the layout of legend component is horizontal or vertical. 布局方式是横还是竖。 [default:Orient....
static Legend defaultLegend
一个在顶部居中显示的默认图例。
void OnChanged()
属性变更时更新textAnchor,minAnchor,maxAnchor,pivot
Dictionary< string, LegendItem > buttonList
the button list of legend. 图例按钮列表。
string formatter
Legend content string template formatter. Support for wrapping lines with . Template:{name}....
float itemHeight
Image height of legend symbol. 图例标记的图形高度。 [default:12f]
void OnChanged()
Callback handling when parameters change. 参数变更时的回调处理。
float runtimeHeight
运行时图例的总高度
@ Rect
正方形。可通过设置itemStyle的cornerRadius变成圆角矩形。
bool itemAutoColor
Whether the legend symbol matches the color automatically. 图例标记的图形是否自动匹配颜色。 [default:true]
void RemoveData(string name)
Removes the legend with the specified name. 移除指定名字的图例。
void SetButton(string name, LegendItem item, int total)
Bind buttons to legends. 给图例绑定按钮。
void RemoveButton()
Remove all legend buttons. 移除所有图例按钮。
int GetIndex(string legendName)
Gets the index of the specified legend. 获得指定图例的索引。
void UpdateContentColor(string name, Color color)
Update the text color of legend. 更新图例文字颜色。
Type iconType
Type of legend. 图例类型。 [default:Type.Auto]
Sprite GetIcon(int index)
Gets the legend button for the specified index. 获得指定索引的图例按钮。
float runtimeEachHeight
单列高度
float itemWidth
Image width of legend symbol. 图例标记的图形宽度。 [default:24f]
TextStyle textStyle
the style of text. 文本样式。
override bool vertsDirty
图表是否需要刷新(图例组件不需要刷新图表)