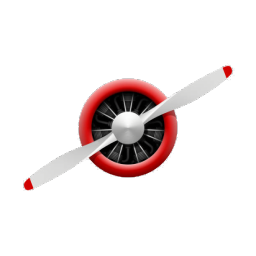 |
AirControl
1.3.0
Open Source, Modular, and Extensible Flight Simulator For Deep Learning Research
|
8 using System.Collections;
10 using UnityEngine.EventSystems;
14 [DisallowMultipleComponent]
18 private Serie serie, serie1;
19 private float m_RadiusSpeed = 100f;
20 private float m_CenterSpeed = 1f;
27 private void OnEnable()
35 StartCoroutine(PieDemo());
40 StartCoroutine(PieAdd());
41 yield
return new WaitForSeconds(2);
42 StartCoroutine(PieShowLabel());
43 yield
return new WaitForSeconds(4);
44 StartCoroutine(Doughnut());
45 yield
return new WaitForSeconds(3);
46 StartCoroutine(DoublePie());
47 yield
return new WaitForSeconds(2);
48 StartCoroutine(RosePie());
49 yield
return new WaitForSeconds(5);
55 chart = gameObject.GetComponent<
PieChart>();
56 if (chart ==
null) chart = gameObject.AddComponent<
PieChart>();
81 chart.
onPointerClickPie = delegate(PointerEventData e,
int serieIndex,
int dataIndex){
84 yield
return new WaitForSeconds(1);
87 IEnumerator PieShowLabel()
93 yield
return new WaitForSeconds(1);
97 yield
return new WaitForSeconds(1);
101 yield
return new WaitForSeconds(1);
105 yield
return new WaitForSeconds(1);
110 IEnumerator Doughnut()
116 serie.
radius[0] += m_RadiusSpeed * Time.deltaTime;
122 yield
return new WaitForSeconds(1);
124 serie.
data[0].selected =
true;
126 yield
return new WaitForSeconds(1);
129 serie.
data[0].selected =
false;
131 yield
return new WaitForSeconds(1);
134 IEnumerator DoublePie()
141 chart.
AddData(1, 1548,
"搜索引擎");
149 serie1.
radius[1] += m_RadiusSpeed * Time.deltaTime;
162 yield
return new WaitForSeconds(1);
165 IEnumerator RosePie()
175 for (
int i = 0; i < 2; i++)
187 while (serie.
center[0] > 0.25f || serie1.
center[0] < 0.7f)
189 if (serie.
center[0] > 0.25f) serie.
center[0] -= m_CenterSpeed * Time.deltaTime;
190 if (serie1.
center[0] < 0.7f) serie1.
center[0] += m_CenterSpeed * Time.deltaTime;
194 yield
return new WaitForSeconds(1);
195 while (serie.
radius[0] > 3f)
197 serie.
radius[0] -= m_RadiusSpeed * Time.deltaTime;
198 serie1.
radius[0] -= m_RadiusSpeed * Time.deltaTime;
208 yield
return new WaitForSeconds(1);
Orient
the layout is horizontal or vertical. 垂直还是水平布局方式。
Position position
The position of label. 标签的位置。
string text
The main title text, supporting for newlines. 主标题文本,支持使用 换行。
TextStyle textStyle
the sytle of text. 文本样式。
RoseType
Whether to show as Nightingale chart, which distinguishs data through radius. 是否展示成南丁格尔图,通过半径区分数据大小。
virtual SerieData AddData(string serieName, double data, string dataName=null)
Add a data to serie. If serieName doesn't exist in legend,will be add to legend. 添加一个数据到指定的系列中。
Location type. Quick to set the general location. 位置类型。通过Align快速设置大体位置,再通过left,right,top,bottom微调具体位置...
SerieType
the type of serie. 系列类型。
void RefreshChart()
Redraw chart in next frame. 在下一帧刷新图表。
RoseType pieRoseType
Whether to show as Nightingale chart. 是否展示成南丁格尔图,通过半径区分数据大小。
SerieLabel label
Text label of graphic element,to explain some data information about graphic item like value,...
bool border
Whether to show border. 是否显示边框。
bool show
Whether to show legend component. 是否显示图例组件。
LineType lineType
the type of visual guide line. 视觉引导线类型。
Location location
The location of legend. 图例显示的位置。 [default:Location.defaultTop]
bool show
Whether the label is showed. 是否显示文本标签。
float top
Distance between component and the left side of the container. 离容器上侧的距离。
string subText
Subtitle text, supporting for for newlines. 副标题文本,支持使用 换行。
Orient orient
Specify whether the layout of legend component is horizontal or vertical. 布局方式是横还是竖。 [default:Orient....
List< SerieData > data
系列中的数据内容数组。SerieData可以设置1到n维数据。
系列。每个系列通过 type 决定自己的图表类型。
float left
Distance between component and the left side of the container. 离容器左侧的距离。
Text label of chart, to explain some data information about graphic item like value,...
float itemHeight
Image height of legend symbol. 图例标记的图形高度。 [default:12f]
Color color
the color of text. 文本的颜色。 [default: Color.clear]
virtual Serie AddSerie(SerieType type, string serieName=null, bool show=true, bool addToHead=false)
Add a serie to serie list. 添加一个系列到系列列表中。
void RefreshLabel()
刷新文本标签Label,重新初始化,当有改动Label参数时手动调用改接口
Legend? legend
The legend setting of chart. 图例组件
float[] center
the center of chart. 中心点。
Action< PointerEventData, int, int > onPointerClickPie
the callback function of click pie area. 点击饼图区域回调。参数:PointerEventData,SerieIndex,SerieDataIndex
Title? title
The title setting of chart. 标题组件
float itemWidth
Image width of legend symbol. 图例标记的图形宽度。 [default:24f]
int fontSize
font size. 文本字体大小。 [default: 18]
Position
The position of label. 标签的位置。
float pieSpace
the space of pie chart item. 饼图项间的空隙留白。
float[] radius
the radius of chart. 半径。radius[0]表示内径,radius[1]表示外径。
virtual void RemoveData()
Remove all data from series and legend. The series list is also cleared. 清除所有系列和图例数据,系列的列表也会被清除。