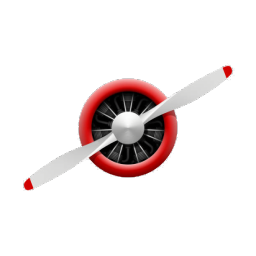 |
AirControl
1.3.0
Open Source, Modular, and Extensible Flight Simulator For Deep Learning Research
|
13 [DisallowMultipleComponent]
18 private float updateTime;
31 if (Input.GetKeyDown(KeyCode.Space))
48 var rate = Random.Range(1, 4);
49 for (
int i = 0; i <= 360; i++)
51 var t = i / 180f * Mathf.PI;
52 var r = Mathf.Sin(2 * t) * Mathf.Cos(2 * t) * rate;
53 chart.
AddData(0, Mathf.Abs(r), i);
float startAngle
Starting angle of axis. 90 degrees by default, standing for top position of center....
virtual SerieData AddData(string serieName, double data, string dataName=null)
Add a data to serie. If serieName doesn't exist in legend,will be add to legend. 添加一个数据到指定的系列中。
SerieType
the type of serie. 系列类型。
float min
The minimun value of axis.Valid when minMaxType is Custom 设定的坐标轴刻度最小值,当minMaxType为Custom时有效。
Tooltip? tooltip
The tooltip setting of chart. 提示框组件
AxisType type
the type of axis. 坐标轴类型。
The axis in rectangular coordinate. 直角坐标系的坐标轴组件。
float max
The maximum value of axis.Valid when minMaxType is Custom 设定的坐标轴刻度最大值,当minMaxType为Custom时有效。
virtual Serie AddSerie(SerieType type, string serieName=null, bool show=true, bool addToHead=false)
Add a serie to serie list. 添加一个系列到系列列表中。
AxisMinMaxType minMaxType
the type of axis minmax. 坐标轴刻度最大最小值显示类型。
AngleAxis? angleAxis
Angle axis of Polar Coordinate. 极坐标系的角度轴。
AxisType
the type of axis. 坐标轴类型。
AxisMinMaxType
the type of axis min and max value. 坐标轴最大最小刻度显示类型。
virtual void RemoveData()
Remove all data from series and legend. The series list is also cleared. 清除所有系列和图例数据,系列的列表也会被清除。